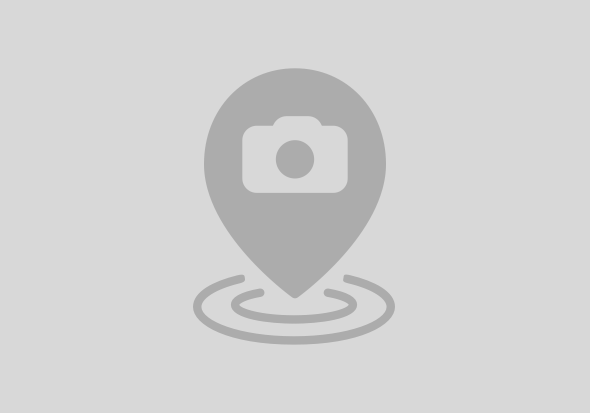
sap.m.App
control for supporting navigation. A List view that consists of a sap.m.Page
control that contains a sap.m.Table
control that renders the appropriate entity set using a sap.m.ColumnListItem
control of type sap.m.ListType.Navigation
.sap.m.routing.Router
class means we can navigate backwards and forwards using the browser buttons and we can do a browser refresh at any time and be returned to the same view and context.sap.tnt.Toolpage
to provide a menu system with an entry for each of the three components built earlier. The ToolPage control contains its' own sap.m.App
control which will be used as a navigation container for the components. The components could be located anywhere, including in their own library, but for this example I simply placed them into a "reuse" subdirectory.{
...
"sap.ui5": {
"rootView": {
"viewName": "yelcho.mydemo.comprouting.view.App",
"type": "XML",
"async": true,
"id": "app"
},
"componentUsages": {
"suppliersComponent": {
"name": "yelcho.reuse.suppliers",
"settings": {},
"componentData": {},
"lazy": true
},
"categoriesComponent": {
"name": "yelcho.reuse.categories",
"settings": {},
"componentData": {},
"lazy": true
},
"productsComponent": {
"name": "yelcho.reuse.products",
"settings": {},
"componentData": {},
"lazy": true
}
},
...
"routing": {
...
"routes": [
{
"name": "home",
"pattern": "",
"target": "home"
},
{
"name": "suppliers",
"pattern": "suppliers",
"target": {
"name": "suppliers",
"prefix": "s"
}
},
{
"name": "categories",
"pattern": "categories",
"target": {
"name": "categories",
"prefix": "c"
}
},
{
"name": "products",
"pattern": "products",
"target": {
"name": "products",
"prefix": "p"
}
}
],
"targets": {
"home": {
"viewId": "home",
"viewName": "Home"
},
"suppliers": {
"type": "Component",
"usage": "suppliersComponent"
},
"categories": {
"type": "Component",
"usage": "categoriesComponent"
},
"products": {
"type": "Component",
"usage": "productsComponent"
},
...
}
}
}
}
sap.ui5.componentUsages
sectionsap.ui5.componentUsages
section.#/suppliers
(which matches the pattern defined in my manifest) and the Suppliers component is loaded.#/suppliers&/s/detail/2
. "s" is the alias defined for the suppliers target so the '/detail/2
' section of the hash is passed to the component router which triggers navigation to the Detail view of the Product component and binds to product 2.sap.m.Table
control that shows the products provided by the current supplier. "routes": [
...
{
"name": "products",
"pattern": "products/{id}",
"target": {
"name": "products",
"prefix": "p"
}
},
...
],
#/products/4
the subcomponent router sees an empty pattern and, in our example, loads the List view because it matches that pattern. "routes": [
{
"name": "list",
"pattern": "",
"target": "list"
},
...
onInit: function() {
Controller.prototype.onInit.apply(this, arguments)
this.getOwnerComponent()
.getRouter()
.getRoute("list")
.attachPatternMatched(this._onPatternMatched, this)
},
_onPatternMatched: function() {
Controller.prototype.onInit.apply(this, arguments)
const oRouter = this.getOwnerComponent().getRouter()
try {
const aHash = oRouter.oHashChanger.parent.hash.split("/")
if (aHash.length > 1) {
switch (aHash[0]) {
case "products":
oRouter.navTo(
"detail",
{
id: aHash[1]
},
true
)
break
default:
}
}
} catch {}
},
...
#/suppliers&/s/products/31&/s-p/categories/4&/s-p-c/products/59&/s-p-c-p/suppliers/28&/s-p-c-p-s/detail/28
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
10 | |
8 | |
5 | |
4 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 |