Beginners Guide to the Java Programming Language
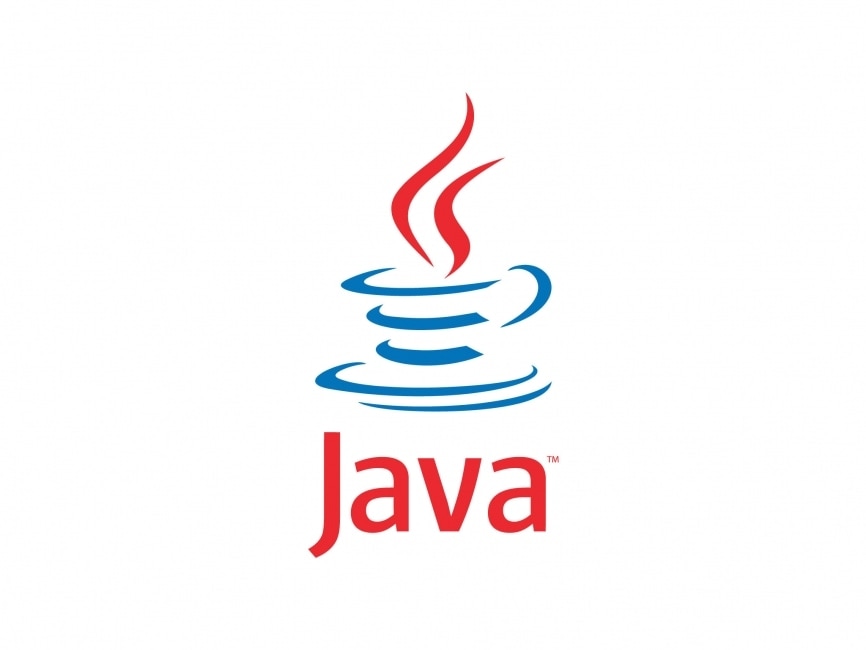
A Beginner’s Guide to Learn Java Programming Language
What Is Java?
Understanding the Basics
Java is a popular, high-level programming language known for its versatility and user-friendly nature. It was created by Sun Microsystems in 1995 and has since become one of the most widely used programming languages in the world. Java is particularly renowned for its “write once, run anywhere” capability, meaning that Java programs can run on any device that has a Java Virtual Machine (JVM).
Key Features of Java
Java offers a wide range of features that make it an ideal choice for beginners. It is a class-based, object-oriented language with a strong emphasis on simplicity and portability. Java’s automatic memory management and built-in security features also contribute to its appeal. Additionally, Java boasts a vast standard library that provides ready-to-use components for various programming tasks.
Introduction to Java language & History of Java
Java, originally developed by Sun Microsystems in the mid-1990s, quickly rose to fame as one of the most popular programming languages in the world. This object-oriented language was designed to be versatile, platform-independent, and easy to use. With its extensive library of functions and tools, Java became the go-to choice for developers across various industries.
Java Versions
Java versions have undergone significant changes over the years, each bringing new features and improvements to the table. From the introduction of lambdas and streams in Java 8 to the module system in Java 9, the updates have aimed to enhance developer productivity and code readability. With every new version, Java evolves to meet the changing needs of the programming world and make our coding experience more efficient and enjoyable. So, whether you’re exploring the latest enhancements in Java 17 or revisiting the classics, embracing these changes can open up a world of possibilities for your projects and coding adventures!
- Java 1.0, released in 1996, was the first version of Java, making it a pioneering milestone in the world of programming languages.
- Java 1.2 (December 1998): Introduced the “assert” keyword for assertion testing.
- Java 5 (September 2004): Brought substantial enhancements with features like generics, enums, annotations, autoboxing, and the enhanced “for” loop.
- Java 7 (July 2011): Added features like the “try-with-resources” statement for improved resource handling and the “diamond operator” for simplifying generic instantiation.
- Java 8 (March 2014): One of the most significant updates, featuring lambda expressions, the Stream API for working with sequences of elements, and the Date and Time API.
- Java 9 introduced the module system, enhancing code maintainability and enabling better organization of large-scale applications.
- Java 10 came with local-variable type inference, making code more concise and readable by allowing the compiler to determine variable types automatically.
- Java 11 marked the end of commercial support from Oracle, switching to a more frequent release schedule with LTS versions every three years.
- Java 14 brought pattern matching for instanceof, simplifying code and enhancing readability when working with complex conditional logic.
- Java 16 introduced records, providing a more concise way to create classes that store data without the need for boilerplate code.
These changes reflect Java’s commitment to staying relevant and user-friendly in the fast-paced world for Java programmers. It’s always exciting to see how Java continues to adapt and innovate with each new version!
How is Java used for real-world applications?
Java is a versatile and powerful programming language that is used in a wide variety of real-world applications. From mobile apps to enterprise systems, Java is the go-to choice for developers around the world. In this article, we will explore how Java is used in real-world applications and why it remains one of the most popular programming languages in the industry.
Java Programming Language Used For Mobile Applications
One of the most common uses of Java in the real world is mobile app development. Android, the most popular mobile operating system, is built on Java. Developers use Java to create robust and user-friendly mobile applications that run seamlessly on a wide range of devices.
Why Use Java for Your Next Project?
When it comes to choosing a programming language for your next project, Java offers a wide range of benefits. From its robust security features to its compatibility with multiple platforms, Java provides developers with the tools they need to create powerful, dynamic applications. Whether you’re a beginner or an experienced programmer, the user-friendly syntax and extensive support for java make it a top choice for any project.
What is the JRE (Java Runtime Environment)?
Java runtime environment, commonly referred to as JRE, is like the engine that powers Java software on your computer. It’s the behind-the-scenes wizard that brings Java programs to life when you double-click on that icon. Think of it as the magic sauce that makes all the Java code you write actually run smoothly. JRE handles the nitty-gritty details, like executing code, managing memory, and handling security, so you can focus on crafting your amazing Java programs without getting bogged down in the technical stuff. So, next time you fire up a Java application and it works like a charm, give a little nod of appreciation to your trusty JRE quietly working its magic in the background.
Getting Started with Java
Setting Up Your Environment
Before you start coding in Java, you need to set up your development environment. This typically involves installing the Java Development Kit (JDK), which includes the Java compiler and other essential tools, as well as an Integrated Development Environment (IDE) such as Eclipse or IntelliJ IDEA.
Learning the Basics
As a beginner, it’s important to grasp the fundamental concepts of Java. This includes understanding variables, data types, operators, control structures, and methods. Familiarizing yourself with these core elements will lay a solid foundation for your journey into Java programming.
Writing Your First Java Program
Once you have a good grasp of the basics, you can dive into writing your first Java program. This often begins with a simple “Hello, World!” program, which serves as an introduction to the syntax and structure of Java code. From there, you can gradually explore more complex programming tasks and concepts.
Program in Java – basic Java tutorials
Hello World in Java
public class HelloWorld {
public static void main(String[] args) {
// This is a simple program that prints “Hello, World!” to the console
System.out.println(“Hello, World!”);
}
}
- This program defines a class named
HelloWorld
. - Inside the class, there’s a
main
method, which is the entry point for Java programs. - The
System.out.println
statement is used to print “Hello, World!” to the console.
Variables and Data Types
public class VariablesExample {
public static void main(String[] args) {
// Declaring variables of different data types
int age = 25; // Integer data type
double salary = 3500.50; // Double data type
char gender = ‘M’; // Character data type
boolean isEmployed = true; // Boolean data type
// Printing out the variables
System.out.println(“Age: ” + age);
System.out.println(“Salary: ” + salary);
System.out.println(“Gender: ” + gender);
System.out.println(“Employed: ” + isEmployed);
}
}
- This program demonstrates the declaration and initialization of variables.
- It includes variables of different data types:
int
,double
,char
, andboolean
. - Each variable is assigned a value, and then they are printed to the console using
System.out.println
.
Conditional Statements (if-else)
public class ConditionalExample {
public static void main(String[] args) {
int num = 10;
// Checking if the number is positive, negative, or zero
if (num > 0) {
System.out.println(num + ” is a positive number.”);
} else if (num < 0) {
System.out.println(num + ” is a negative number.”);
} else {
System.out.println(num + ” is zero.”);
}
}
}
- This program demonstrates the use of
if
,else if
, andelse
statements for conditional branching. - Depending on the value of
num
, it prints whether the number is positive, negative, or zero.
Loops (for loop)
public class LoopExample {
public static void main(String[] args) {
// Using a for loop to print numbers from 1 to 5
for (int i = 1; i <= 5; i++) {
System.out.println(“Number: ” + i);
}
}
}
- This program showcases a simple
for
loop that iterates from 1 to 5. - Inside the loop, it prints each number along with the message “Number: “.
Arrays
public class ArrayExample {
public static void main(String[] args) {
// Creating an array of integers
int[] numbers = {10, 20, 30, 40, 50};
// Accessing and printing elements of the array
for (int i = 0; i < numbers.length; i++) {
System.out.println(“Element at index ” + i + “: ” + numbers[i]);
}
}
}
- This program demonstrates the creation and usage of an array in Java.
- An array named
numbers
is created with five elements. - It then uses a
for
loop to iterate through the array and prints each element along with its index.
Methods
public class MethodExample {
public static void main(String[] args) {
int result = addNumbers(5, 10);
System.out.println(“Result: ” + result);
}
// A method to add two numbers and return the result
public static int addNumbers(int a, int b) {
return a + b;
}
}
- This program illustrates the creation and usage of a method in Java.
- The
addNumbers
method takes two integers as parameters, adds them, and returns the result. - In the
main
method, it callsaddNumbers
with arguments5
and10
, then prints the result.
Object-Oriented Programming (Classes and Objects)
// Define a Car class
class Car {
String brand;
String model;
int year;
// Constructor for Car class
public Car(String brand, String model, int year) {
this.brand = brand;
this.model = model;
this.year = year;
}
// Method to display car information
public void displayInfo() {
System.out.println(“Brand: ” + brand);
System.out.println(“Model: ” + model);
System.out.println(“Year: ” + year);
}
}
public class CarExample {
public static void main(String[] args) {
// Creating objects of the Car class
Car car1 = new Car(“Toyota”, “Camry”, 2020);
Car car2 = new Car(“Ford”, “Mustang”, 2019);
// Calling the displayInfo method for each car
System.out.println(“Car 1:”);
car1.displayInfo();
System.out.println(“\nCar 2:”);
car2.displayInfo();
}
}
- This program demonstrates object-oriented programming concepts in Java.
- It defines a
Car
class with attributesbrand
,model
, andyear
, along with a constructor and a methoddisplayInfo
to print car information. - In the
main
method, twoCar
objects (car1
andcar2
) are created with different values, and then their information is displayed using thedisplayInfo
method.
These examples cover some fundamental aspects of Java programming, from basic syntax to object-oriented concepts.
Advantages of Learning Java
Versatility and Compatibility
Java’s ability to run on various platforms and devices makes it an incredibly versatile language. Whether you’re developing web applications, mobile apps, enterprise software, or embedded systems, Java can accommodate a wide range of programming needs.
Abundance of Resources
As a popular and well-established language, Java offers a wealth of resources for learning and support. There are numerous tutorials, documentation, community forums, and libraries available to help beginners navigate their Java learning journey.
Career Opportunities
Proficiency in Java opens up a multitude of career opportunities in the software development industry. Many companies and organizations actively seek Java developers, making it a valuable skill to possess in today’s job market.
In conclusion, Java is an accessible and powerful programming language that offers a multitude of benefits for beginners. By learning Java, you can embark on a fulfilling journey into the world of software development, equipped with a versatile skillset and abundant resources to support your growth.
How Does Java Compare to Other Programming Languages?
When compared to other popular programming languages like JavaScript or C++, Java stands out for its simplicity, readability, and scalability. With its extensive library of tools and functions, Java offers developers the flexibility to create a wide range of applications, from simple programs to complex enterprise solutions.
Java and JavaScript: Demystifying the Differences
Despite their similar names, Java and JavaScript are fundamentally different languages:
- Java: A statically-typed, compiled language primarily used for backend development, Android apps, and enterprise systems.
- JavaScript: A dynamically-typed, interpreted language used for frontend web development, adding interactivity to websites.
While they share some syntax similarities, their purposes and ecosystems are distinct. Understanding these differences is crucial for aspiring developers deciding which language to learn.
Read more What Is Javascript (JS)
Java Best Practices & Coding Tips
To make the most of your Java programming experience, it’s important to follow best practices and coding tips. By writing clean, efficient code and following industry standards, you can ensure that your Java projects are well-structured, maintainable, and error-free.
The Future of Java Technology – What Lies Ahead?
As technology evolves, Java continues to adapt and innovate. Some trends and developments to watch for include:
- Java 21: The latest versions of Java introduce new features and enhancements, improving performance and security.
- Cloud-Native Java: With the rise of cloud computing, Java is being optimized for cloud-native development, offering scalability and flexibility.
- Microservices Architecture: Java is well-suited for microservices, allowing developers to build modular, independently deployable services.
- Machine Learning and AI: Java libraries like Deeplearning4j are making strides in the field of machine learning and artificial intelligence.
Summary: What You Need to Remember
- Java is a versatile, object-oriented programming language with a rich history and wide range of applications.
- Learning Java is easy with a variety of resources available to help you get started.
- Java’s core features, such as its runtime environment and extensive APIs, make it a top choice for developers.
- By following best practices and coding tips, you can ensure that your Java projects are successful and error-free.
In conclusion, Java is a powerhouse programming language that continues to play a crucial role in the developer community. Whether you’re a beginner looking to learn the basics or an experienced pro tackling complex projects, Java has the tools and support you need to succeed. So why wait? Start your Java journey today and unleash your full coding potential!
FAQs
Q: What is Java programming language?
A: Java is a programming language and computing platform that was developed by Sun Microsystems (now owned by Oracle Corporation) in 1995. It is designed to be platform independent, allowing developers to write code once and run it on any device that supports Java.
Q: How does Java work?
A: Java code is compiled into bytecode, which is then interpreted and executed by the Java Virtual Machine (JVM). The JVM translates the bytecode into machine code that can be understood by the underlying operating system.
Q: What is a Java developer?
A: A Java developer is a software engineer who specializes in writing applications using the Java programming language. They are responsible for designing, developing, and maintaining Java-based applications for various platforms.
Q: What is Java platform?
A: The Java platform is a software environment that provides the necessary tools and libraries for developing and running Java applications. It includes the Java Development Kit (JDK) for compiling and debugging code, as well as the Java Runtime Environment (JRE) for running Java applications.
Q: What is Java API?
A: The Java API (Application Programming Interface) is a set of pre-written code libraries that provide commonly used functions and classes for Java developers. It simplifies the process of developing applications by offering ready-made solutions for common programming tasks.
Q: How to run Java programs?
A: To run Java programs, you need to have the Java Runtime Environment (JRE) installed on your system. Once installed, you can execute Java applications by running the ‘java’ command followed by the name of the Java class that contains the ‘main’ method.
Q: What is a Java class?
A: In Java, a class is a blueprint for creating objects. It defines the properties and behaviors that an object of that class will have. Classes are the building blocks of Java programs and are used to model real-world entities or concepts.
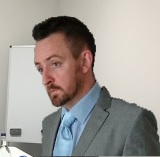
I am a self-motivated, passionate website designer and developer. I have over ten years’ experience in building websites and have developed a broad skill set including web design, frontend and backend development, and SEO.
Using my growing knowledge base I have built my own company (scriptedart.co.uk) creating websites and ecommerce stores and producing custom graphics and web app functionality for a range of local businesses.