What is Instantiation in Java?
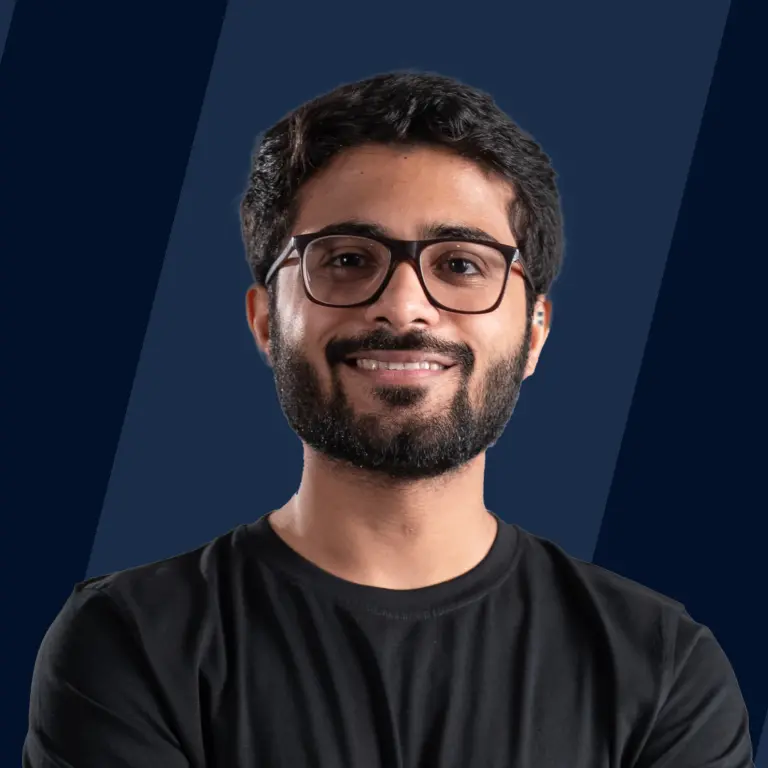
Java uses instantiation to create new instances or objects that are used within the program.
By instantiation, we mean the act of calling a class's constructor, which creates an instance or object of that class. During this process, memory is allocated for the object, and a reference to it is returned. Essentially, the class acts as a blueprint, and instantiation produces an object based on this blueprint.
Now, what exactly do we mean by an object here?
An object is a runtime entity derived from a class. It holds the actual data or state, represented by attributes, and can perform actions, represented by methods. The object is essentially a real-world representation of a class blueprint. With Java's object-oriented capabilities, we can create multiple instances of a class to represent diverse user-defined data structures such as vectors, lists, and more.
Since we know that a class provides the blueprint for objects thus we can create an object from a class. Let's see an example of the CreateObjectDemo program here which will show us how can object is created and then assigned to a variable
Let's break it line by line to understand it better
Here an object is created of the Point class
In these two lines we can see an object is created of rectangle class with different arguments Let's discuss in detail what the above lines of code imply here:
- Declaration: The code set in bold are all variable declarations that associate a variable name with an object type
- Instantiation: For creating an object in Java we use the new keyword which is a java operator
- Initialization: Shortly after the new operator, the constructor is called for initializing the new object We'll learn more about this further in this article
Let's take a brief look at what we mean by classes here
A class is a group of objects which have common properties. It is a template or blueprint from which objects are created. It is a logical entity. It can't be physical.
A class in Java can contain:
- Fields
- Methods
- Constructors
- Blocks
- Nested class and interface
Syntax to declare a class:
Syntax for Instantiation
Let's see the syntax of Instantiation in Java
Another way of writing this syntax in Java is
Creating Instances (Instantiation of an Object in Java)
In Java programming process of creating the object of a class is referred to as Instantiation. The initial memory space of an object is then occupied and a reference is then returned Object instantiation is provided by the blueprint of the class and an unlimited number of class objects are created for representing user-defined data such as lists
Two ways to create instances are:
- Using the new Keyword
- Using Static Factory Method
Using the new Keyword
In Java, the new keyword plays a crucial role in the instantiation process, allowing us to create objects or instances of a class. The term "instantiation" refers to the process of allocating memory for a new object and initializing it using the class constructor. This is why objects are often referred to as instances of their corresponding classes.
Let's delve into a practical example:
Alternatively, we can declare and instantiate the class in one line:
When we use the new keyword followed by a class constructor, it results in the creation of a new instance of that class.
Using Static Factory Method
A static factory method can be used to instantiate a class. A class can provide a static method that returns an instance of the class, which acts as a public static factory method. Remember, this differs from the factory method pattern.
Providing a static factory method instead of a constructor has both advantages and disadvantages.
Advantages of the static factory method:
- Unlike constructors, static factory methods have names, making their purpose clearer.
- Static factory methods don't always need to create a new object every time they're invoked.
- They can return an object of any subtype of their return type, unlike constructors.
- They reduce the verbosity of creating parameterized type instances.
Disadvantages of static factory methods:
- Classes without public or protected constructors cannot be sub-classed.
- Static factory methods are not readily distinguishable from other static methods, making them less intuitive for some developers.
Example of a static factory method:
Examples
Let’s see some examples of object instantiation in Java.
Example 1: Instantiate a Single Object in Java
We have a class named JavaClass with variables x, y, Sum() and main() method as user-defined and predefined methods.
Output:
An instance or the object of class jc in the main method is created using the new keyword. With this object, we can access the Sum() method, and then the returned value is stored in the ans variable. System.out.println() is utilized for printing out the sum at the console.
Example 2: Instantiate a Single Object in Java Using Multiple Classes
An object of a class can also be created in another class, and public methods of that class can be accessed. Here, in this example, we have two classes: JavaClass1 and Example.
Wherein, JavaClass1 contains a method named Message() and a String type variable name:
Here, an object of class JavaClass1 is created in the main method of class Example and can access all the public methods of JavaClass1 declared in the second class named Example. Using jc, we call the JavaClass1 method in the main method of Example class.
Output:
Example 3: Instantiate Multiple Objects in Java Using Multiple Classes
Multiple instances of the same class can be created. The two classes in this example are the same as those in the one above. In the main function of the second class Example, we will now generate several objects of the class JavaClass1.
In our Javaclass1, a constructor, 2 user-defined methods, and two variables are included. Reference variables to the global variables of the class are assigned in the constructor. Methods like Sum() and Sub() return the sum and differences of variables x and y.
Let's see an example in Java:
Two objects jc and jc1 are created by passing integer values as arguments in the main method of class Example here. Class variables are instantiated by the constructor with the given values. Lastly, the Sum() method and sub() method are accessed using jc and jc1 objects.
Output:
Instantiation of Primitives
Java, being a statically-typed programming language, requires all variables to be declared with a specific type before they can be used. The variable's type determines the values it can hold and the operations that can be performed on it.
Example:
Java provides several predefined primitive types, which are represented by reserved keywords. The primitive types supported by Java include: byte, short, int, long, float, double, boolean, and char.
For fields that are declared but not initialized, the Java compiler provides default values. The exact default value varies based on the primitive type but is typically zero for numeric types or false for the boolean type. It's important to note that this default initialization is provided for class member variables (fields) and not for local variables.
Unlike objects, when a variable of a primitive type is declared and initialized, the new keyword is not used. This is because primitives are fundamental data types built directly into the language, not instances of classes or objects. Literal values, such as 10 in the example above, are used to initialize primitives. These literals represent fixed values in the source code.
Can We Instantiate Abstract Class?
Abstraction is the technique of hiding implementation specifics from the user and only displaying functionality.
In a different method, it just displays the user's needs and hides internal information, such as when sending an SMS, where you write the text and send the message. You are unaware of the internal operations involved in message delivery.
When an object is abstracted, you may concentrate on what it does rather than how it does it.
There are two ways to achieve abstraction in java
- Abstract class (0 to 100%)
- Interface (100%)
No, abstract classes cannot be instantiated. However, they are subclassifiable. Typically, when an abstract class is subclassed, all of the abstract methods in the parent class have implementations.
We've all heard of abstract classes, which are classes that can have abstract methods but cannot be instantiated. Java does not allow us to instantiate abstract classes since they are incomplete and therefore unable to be used.
Abstract Class in Java
An abstract class in Java is one that cannot be instantiated on its own but can be subclassed by other classes. It can contain both abstract (without implementation) and concrete (with implementation) methods.
Example:
Abstract Method in Java
An abstract method is a method that's declared but has no concrete implementation within the abstract class. It's essentially a placeholder meant to be overridden in a subclass.
Example:
Abstract Class with an Abstract Method
Consider the example below:
Output:
Here, Bike is an abstract class with an abstract method named run(). The concrete implementation of this method is provided by the subclass Honda4. When we create an object of Honda4 and call the run() method, it displays "running safely".
Abstract Classes in Java
An abstract class in Java cannot be instantiated on its own. It can, however, be subclassed.
Example 1: Demonstrating Error when Instantiating an Abstract Class
Output
The output demonstrates that the abstract class ClassOne cannot be instantiated.
Example 2: Using Anonymous Classes in Java
Output
In this example, we aren't instantiating the abstract class directly. Instead, we're creating an instance of an anonymous subclass of ClassOne. This demonstrates that abstract classes themselves cannot be instantiated, but their anonymous subclasses can be.
Example 3: Anonymous Class Implementing an Interface
Output
Here, an anonymous class implements the NewClass interface. This example shows that interfaces, like abstract classes, cannot be instantiated directly. However, they can be implemented by anonymous classes.
Example 4: Anonymous Class Implementing an Abstract Class
Output
In this example, an anonymous class provides the implementation for the abstract method implementMethod() of the ClassOne abstract class. This highlights the ability of anonymous classes to implement abstract classes, providing implementations for their abstract methods.
Difference between Instantiation and Initialization
Instantiation and initialization are completely different concepts in Java programming.
Instantiation
Memory is allocated for an object at this point. The new keyword is accomplishing this. The new keyword returns a reference to the newly generated object.
A class can only be instantiated after it has been loaded and initialized (though all methods do not need to have been verified). The class provides the object's size, and that heap is then identified and zeroed. A pointer to the class and other fields needed to handle the class is filled up in the object header. The appropriate constructor method for the class is then called (and any super's constructor will be called at this point)
Initialization
Values are now loaded into the allotted memory at this point. When a class's constructor uses the new keyword, it performs this.
Additionally, a variable must be initialized by being given a reference to an object in memory.
Values are now loaded into the allotted memory at this point. When a class's constructor uses the new keyword, it performs this.
A Java class must initialize the class representation in a number of ways when it is "loaded" into the JVM.
- A runtime structure is expanded from the class's "constant pool" and some of its variables are initialized.
- The constant pool is used to locate the class's superclass and extract its properties. For the class's methods, a method table is created. The various techniques are identified as "not yet confirmed." The class representation undergoes a number of verification processes. It initializes static fields.
- String literals are "interned" upon initial reference, and the interned string pointer is added to the constant pool.
- A variable must also be initialized by having a reference to an object in memory supplied to it.
- On first reference methods are "validated"
Let's see an example of Initialization and Instantiation in Java, together on the same line
Let's now see an example of initializing a variable on a separate line in memory in Java
Learn More
Learn more about abstract classes here
Know more about objects and classes here
Know more about methods in Java here
Conclusion
- Instantiation refers to the process of creating an instance or object of a specific class by invoking its constructor.
- Initialization involves assigning a specific value to a variable or setting initial states for an object.
- Abstract classes in Java cannot be instantiated directly because they may contain abstract methods which lack concrete implementations.
- Given Java's static typing, variables must be declared with their data types before they can be used, ensuring type safety.
- A class serves as a blueprint or template from which objects are created, encapsulating properties and behaviors that are common to all its instances.
- Abstraction promotes hiding the intricate details of how a function is implemented, emphasizing just the essential features or behaviors to the user.
- To instantiate a class, besides constructors, one can use static factory methods. These are static methods that return instances of the class, offering an alternative way to create objects.